digitalmars.D.learn - How do I _really_ implement opApply?
- WebFreak001 (95/95) Nov 29 2022 it seems now when trying to cover scope semantics, @safe/@system
- WebFreak001 (1/1) Nov 29 2022 note: all of these functions are prefixed with `scope:`
- zjh (3/4) Nov 29 2022 Should there be an `intermediate layer` to simplify such function
- zjh (3/5) Nov 29 2022 There should be a `placeholder` similar to `inout` that can
- Steven Schveighoffer (4/8) Nov 29 2022 1. use the template
- Vladimir Panteleev (5/12) Nov 29 2022 +1. I use this pattern often:
it seems now when trying to cover scope semantics, safe/ system and pure it already becomes quite unmanagable to implement opApply properly. Right now this is my solution: ```d private static enum opApplyImpl = q{ int result; foreach (string key, ref value; this.table) { result = dg(key, value); if (result) { break; } } return result; }; public int opApply(scope int delegate(string, ref TOMLValue) safe dg) safe { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) safe dg) safe { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref TOMLValue) safe dg) safe { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) safe dg) safe { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) safe dg) safe const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) safe dg) safe const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref TOMLValue) safe pure dg) safe pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) safe pure dg) safe pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref TOMLValue) safe pure dg) safe pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) safe pure dg) safe pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) safe pure dg) safe pure const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) safe pure dg) safe pure const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref TOMLValue) system dg) system { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) system dg) system { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref TOMLValue) system dg) system { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) system dg) system { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) system dg) system const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) system dg) system const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref TOMLValue) system pure dg) system pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) system pure dg) system pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref TOMLValue) system pure dg) system pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) system pure dg) system pure { mixin(opApplyImpl); } public int opApply(scope int delegate(string, ref const TOMLValue) system pure dg) system pure const { mixin(opApplyImpl); } public int opApply(scope int delegate(string, scope ref const TOMLValue) system pure dg) system pure const { mixin(opApplyImpl); } ``` Surely there is a better way to do this?! Better formatted: 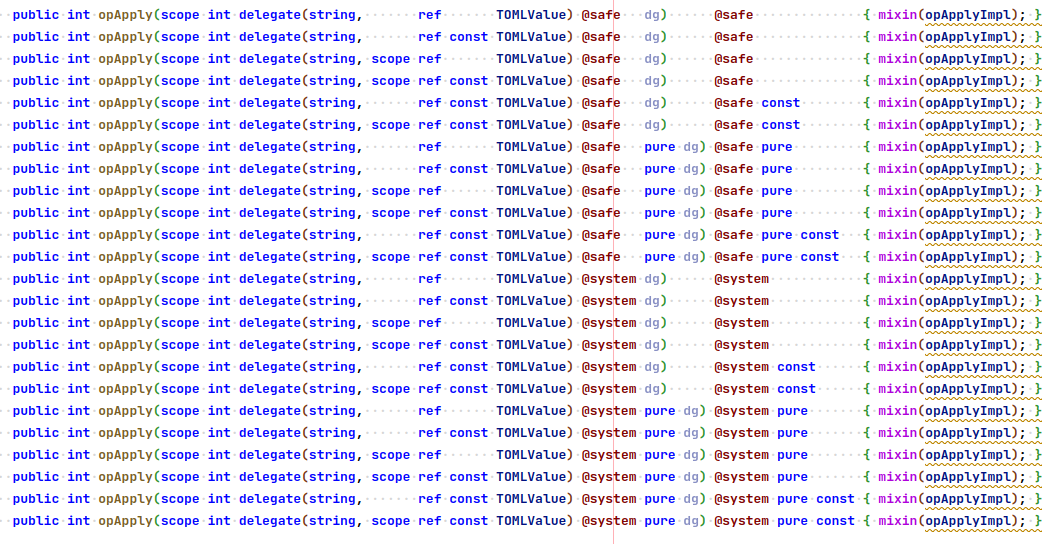 (note: I don't want to use a template, this way of writing it has the advantage that the compiler checks all different code paths for errors, so the errors aren't delayed until someone actually tries to iterate over my data structure)
Nov 29 2022
note: all of these functions are prefixed with `scope:`
Nov 29 2022
On Wednesday, 30 November 2022 at 00:50:46 UTC, WebFreak001 wrote:...Should there be an `intermediate layer` to simplify such function calls?
Nov 29 2022
On Wednesday, 30 November 2022 at 01:17:14 UTC, zjh wrote:Should there be an `intermediate layer` to simplify such function calls?There should be a `placeholder` similar to `inout` that can absorb all `attributes` of the parameter.
Nov 29 2022
On 11/29/22 7:50 PM, WebFreak001 wrote:(note: I don't want to use a template, this way of writing it has the advantage that the compiler checks all different code paths for errors, so the errors aren't delayed until someone actually tries to iterate over my data structure)1. use the template 2. use a unittest to prove they all compile. -Steve
Nov 29 2022
On Wednesday, 30 November 2022 at 01:30:03 UTC, Steven Schveighoffer wrote:On 11/29/22 7:50 PM, WebFreak001 wrote:+1. I use this pattern often: https://github.com/CyberShadow/ae/blob/86b016fd258ebc26f0da3239a6332c4ebecd3215/utils/graphics/libpng.d#L716-L721 https://github.com/CyberShadow/ae/blob/86b016fd258ebc26f0da3239a6332c4ebecd3215/utils/math/combinatorics.d#L220-L222(note: I don't want to use a template, this way of writing it has the advantage that the compiler checks all different code paths for errors, so the errors aren't delayed until someone actually tries to iterate over my data structure)1. use the template 2. use a unittest to prove they all compile.
Nov 29 2022